Mobincube's Javascript SDK
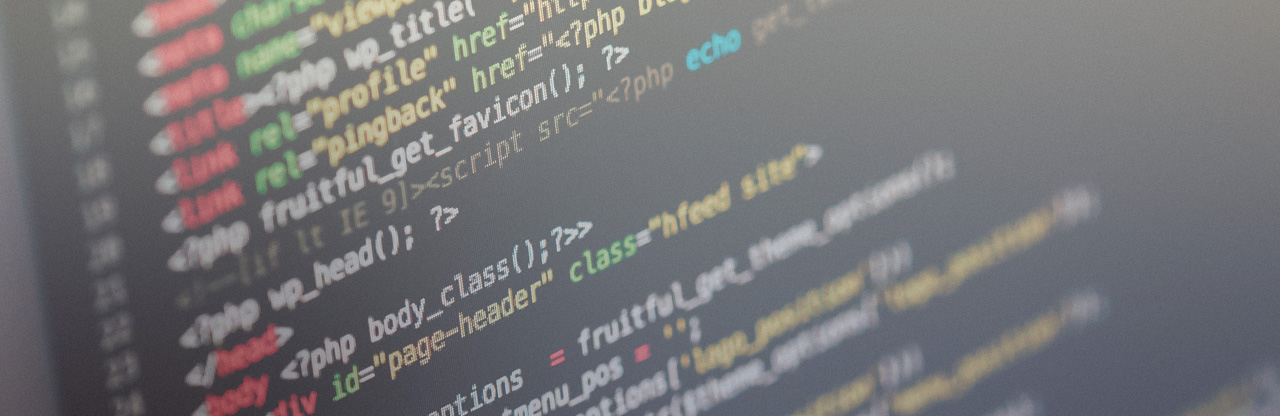
All the properties are stored inside the properties.json file, which uses standard JSON format. You can just load it into memory and start accessing any property from your javascript code.
But the best way to load and access the properties is by integrating our lightweight JS SDK. In the initialization process, all the properties are decoded into a javascript object so that you can access them anytime.
Setting up
Download Mobincube's JS SDK from this link.
Place the file properties.min.js inside your html project.
Include the SDK at the end of the
tag, and before your own script:<script src="js/properties.min.js"></script>
<script src="js/main.js"></script>
</body>
Initializing the SDK
In order to start the SDK and load the properties into memory from the properties.json file, add this code on the top of your javascript code:
success: onPropertiesReady
});
function onPropertiesReady() {
// Add your initialization code here...
}
The function onPropertiesReady (or whatever name you choose for that function) will be automatically called right after all the properties are loaded into memory and are ready to be used in your javascript code. Please make sure that the properties.json file is placed in the root folder of your AddOn.
Use the values of the "properties" object in your scripts in order to customize things.
Behaviour during App runtime
The function onPropertiesReady is called only the first time the screen containing the AddOn is loaded. If the screen is already in the navigation history and you are accessing it by coming back from another screen, it is not reloaded, so the funcion onPropertiesReady won't be called. It doesn't matter if the user is returning to the screen via the action back or the action section.
If you set the screen as a non-anchored screen, it won't be registered in the navigation history and returning to the screen (only possible with the action section) will make the screen be reloaded, and thus the onPropertiesReady function will be invoked.
In case you need to keep the screen as an anchored screen and want to reset some values every time the user accesses it, even if the screen was already loaded, you have to define the function mobincube_onComingFromOtherScreen. This function is automatically invoked every time a user returns to a screen that is already loaded. This might be useful in case you need to restart timers, etc.
// Add your code here
}
Behaviour while editting on Mobincube
When the publisher of Mobincube is editting the properties of your AddOn, it behaves differently as on app's runtime. Basically, the onPropertiesReady function is invoked everytime the publisher edits a property. You can use a flag value to detect whether it is the first time the onPropertiesReady is invoked or not. In case your AddOn generates elements on runtime, you should only do it the first time. You should only change css values all other times when the onPropertiesReady is called. This will make things easier for the publisher to customize your AddOn, since properties changes will be seen instantly on the previsualization area.
function onPropertiesReady() {
if( isFirstTime ){
// Create elements...
isFirstTime = false;
}else{
// Edit element attributes...
}
}
Sometimes the content that you might want to show on the screen while the publisher is editting the App is different to the one you'll show in the real app. For example, in case you want to show instructions on how to use the AddOn. There's an easy way to check if the AddOn is running inside Mobincube's interface. Mobincube uses an <iframe> to load your AddOn on the interface, so its window.parent is not the window itself:
if( !(window === window.parent) ){
// This code will be reached only during app edition...
}else{
// This code will only be reached during app runtime...
}
}
Accessing properties from JS
Once the properties object is ready, accessing any property value is quite straight forward. The properties object contains an array called properties which contains all of them. You can access any property in that array by using its key:
"tabs": [{
"key": "style",
"label": "STYLE",
"properties": [{
"key": "bgColor",
"type": "color",
"label": {"en":"Background color:","es":"Color de fondo:"},
"value": "rgb(0,0,0)",
"format": "hex"
}]
}]
}
var element = document.querySelector("#myDiv");
element.style.backgroundColor = properties.properties.bgColor;
}